Tailwind. Why and why not?
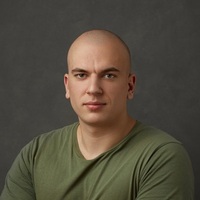
Mileta Dulovic
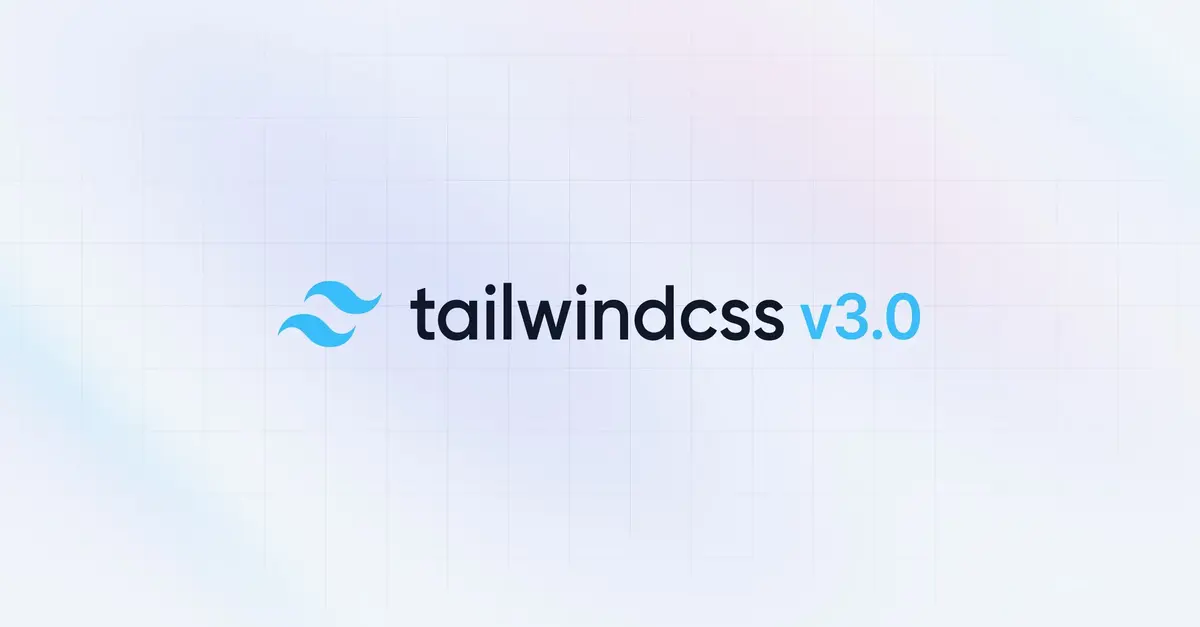
You probably don't need to use it, at least all of it, because readability and code maintenance will most likely suffer.
What is Tailwind
A utility-first CSS framework packed with classes like flex, pt-4, text-center and rotate-90 that can be composed to build any design, directly in your markup.
This means that you get set of predefined classes (or you can create dynamic styles on the fly) that you add to style HTML elements.
Here is an example of changing text color without tailwind
<p class="hello-world">Hello World</p>
.hello-world {
color: red;
}
And here is an example with tailwind.
// using predefined color
<p class="text-red-500">Hello World</p>
// or adding custom color
<p class="text-[#ffffff]">Hello World</p>
As you can see the code is shorter with tailwind because we don't need to write css for class, and even if we do, we can use [] brackets to specify colors,
sizes, and styles.
The thing is that things can easily get out of control, especially if you use pseudo classes like :after
or :before
.
Readability
Consider this piece of code that uses :before
pseudo class to add vertical line on the left side of the text.
<div
class="relative italic before:content-[''] before:w-[4px] before:h-full before:bg-white before:absolute before:top-0 before:left-0 pl-3 ml-2 my-4"
></div>
Now let's write the same thing in CSS
div {
position: relative;
font-style: italic;
}
div:before {
content: "";
width: 4px;
height: 100%;
background: #fff;
position: absolute;
top: 0;
left: 0;
}
These to examples do the same exact thing, just in 2 different ways. I prefer second one much more over the tailwind example, as it is much easier to read.
Performance
Tailwind has great performance. They introduced JIT (Just-In-Time) Mode in version 2.1+, it is really fast, and it allows us to use arbitrary styles without
writing custom CSS
.
Tailwind gives you a huge set of predefined classes to use while developing project. Great thing is that it will remove all unused classes when starting your
project in production mode. This ensures that not even a single byte is wasted on unused code, and it is really handy, because as developers we will definitely
ship some unused code to production (especially CSS
).
Is performance worth it
Performance shouldn't always be the only that we need to take care of. Yes, it is important that we keep our project as smooth as possible,
but is it really important if it means that you can't manage your own code after few months, or if your co-worker has to jump in on the project?
Jake Archibald said, in his show (HTTP 203), that it is sometimes better to sacrifice performance for the readability.
If you think about that for a moment it actually makes a lot of sense when talking about front-end development. With HTML, CSS, JS, and all these
front-end frameworks, shit will definitely hit the fan at one point.
What good is a code that can't be maintained.
When do I use tailwind
I use it on almost every project, and I love creating complex layouts with it. It really takes a lot of work off of my back.
Here is a Tailwind representation of 3 divs in single row on big screens, and stacked rows on mobile phones
<div class="grid grid-cols-1 lg:grid-cols-3">
<div></div>
<div></div>
<div></div>
</div>
Divs will automatically collapse when users is on a small screen, which is really handy. Now here is the same code in CSS
<div class="parent">
<div></div>
<div></div>
<div></div>
</div>
div.parent {
display: grid;
grid-template-columns: repeat(3, minmax(0, 1fr));
}
@media screen and (max-width: 1024px) {
div.parent {
grid-template-columns: repeat(1, minmax(0, 1fr));
}
}
As you can see, in this situation Tailwind is really great and easy to write and maintain.
Conclusion
There is a lot more about tailwind than it was written here. This post was just my look on the things and how I work on my projects. You should definitely visit tailwindcss.com for more information.
A sword is only as good as the man who wields it.