Pure CSS carousel
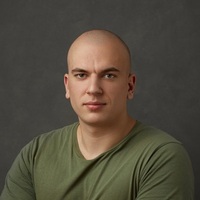
Author
Mileta Dulovic
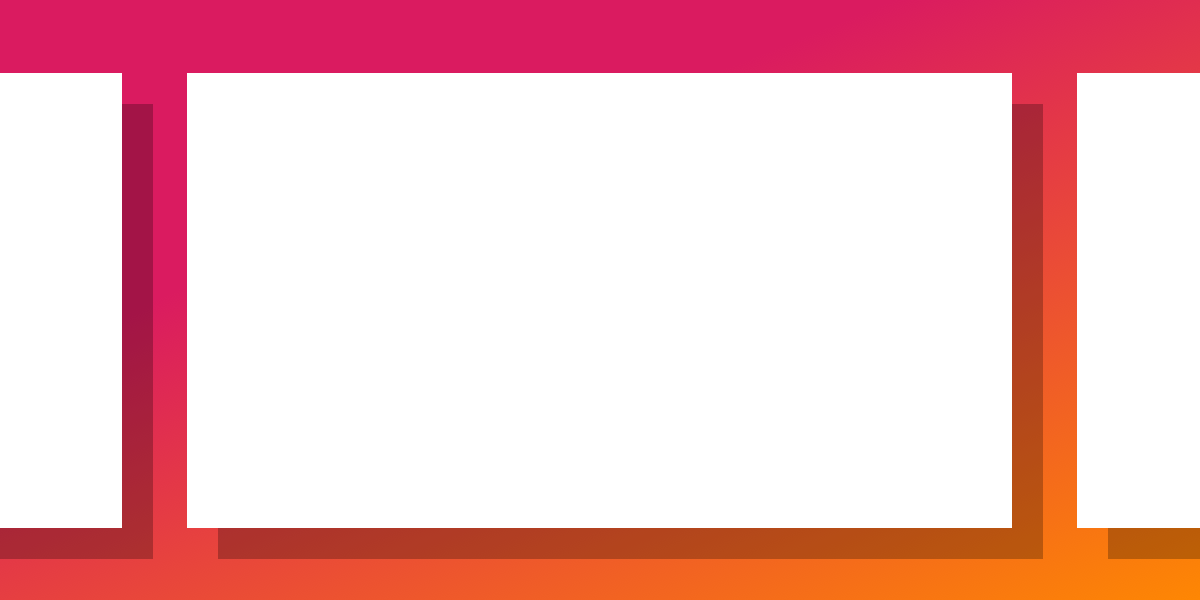
I am not sure if you knew, but you can utilize horizontal scroll
and flexbox
to create awesome carousel
with just CSS
and a bit of HTML
.
Steps
- Set slides in flexbox and don't let them wrap
- Make the parent scrollable
- Line up the slides with
scroll-snap-type
- Use
scroll-snap-stop
to scroll slides 1 by 1
<div class="slides-container">
<div class="slide">1</div>
<div class="slide">2</div>
<div class="slide">3</div>
<div class="slide">4</div>
<div class="slide">5</div>
<div class="slide">6</div>
</div>
.slides-container {
display: flex;
overflow: auto;
flex: none;
flex-flow: row nowrap; /* sets the flex flow to row, and forbid children from wrapping */
scroll-snap-type: x mandatory; /* this allow us to snap the items when they are scrolled */
width: 100%;
gap: 20px;
height: 200px;
}
.slides-container::-webkit-scrollbar {
display: none;
}
.slides-container .slide {
scroll-snap-stop: always; /* with this user scroll slides one by one, and can't skip them */
scroll-snap-align: center;
flex: none;
width: 100%;
height: 100%;
overflow-y: auto;
background-color: skyblue;
display: flex;
align-items: center;
justify-content: center;
font-size: 80px;
font-weight: bold;
color: #fff;
}
Example
Here is the example that you can play with. Try changing code and see what happens.
Note
You can drag this carousel with mouse, as that would require JavaScript
logic.
More in this category
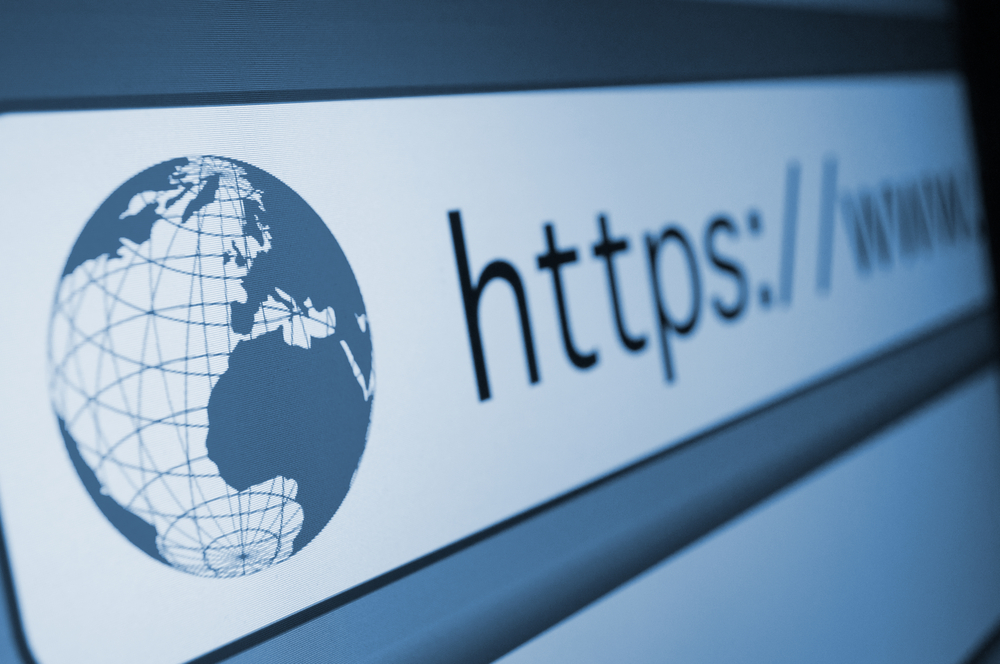
Get any website favicon using free Google API
Google has free API endpoint that allows us to get favicon of any website in different sizes
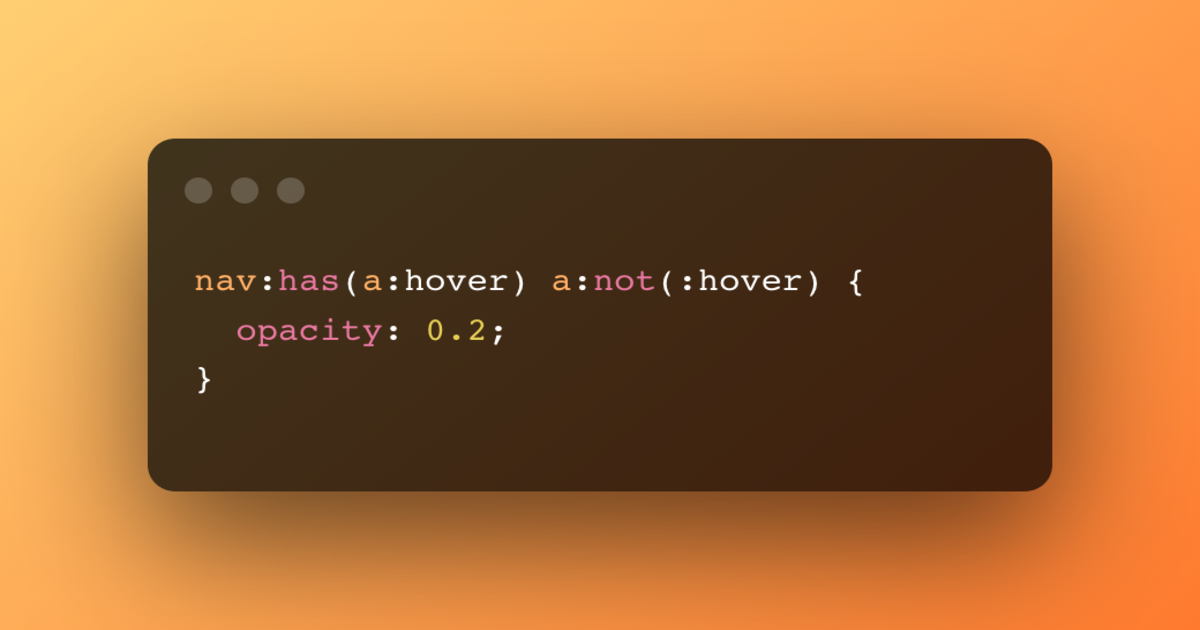
CSS new :has selector
Want to know more about new awesome selector added in CSS? Let us dive right in!
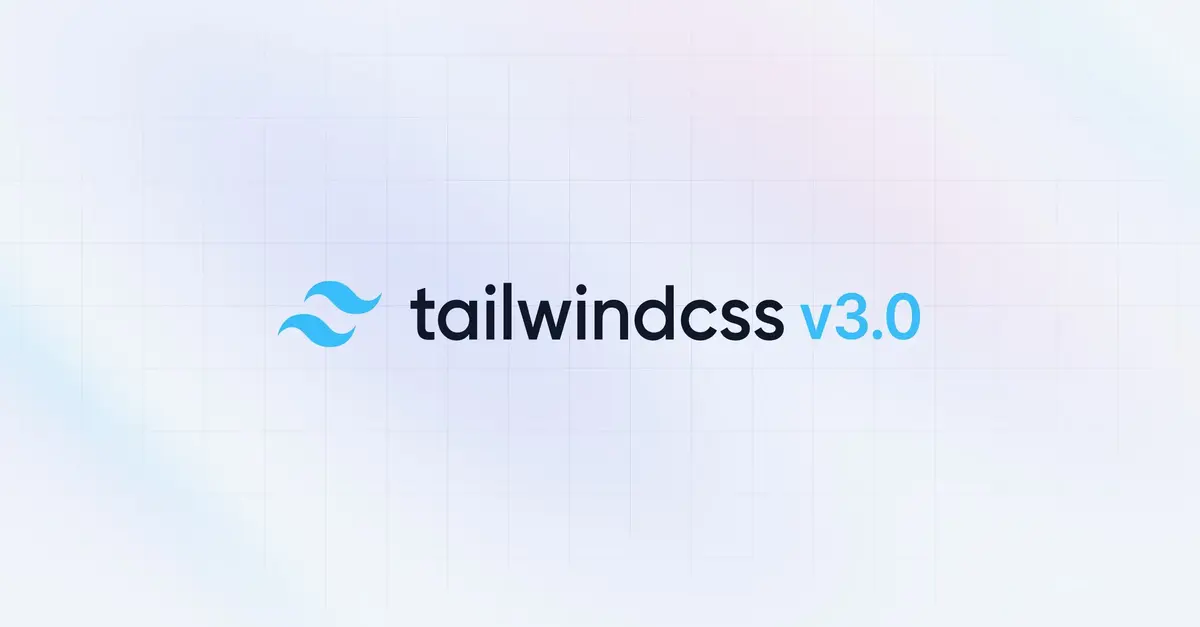
Tailwind. Why and why not?
Let us talk about Tailwind. Do you really need to use it, and does it actually helps you.