JavaScript Loops performance
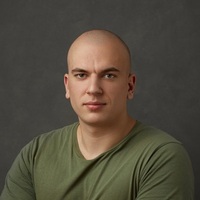
Mileta Dulovic
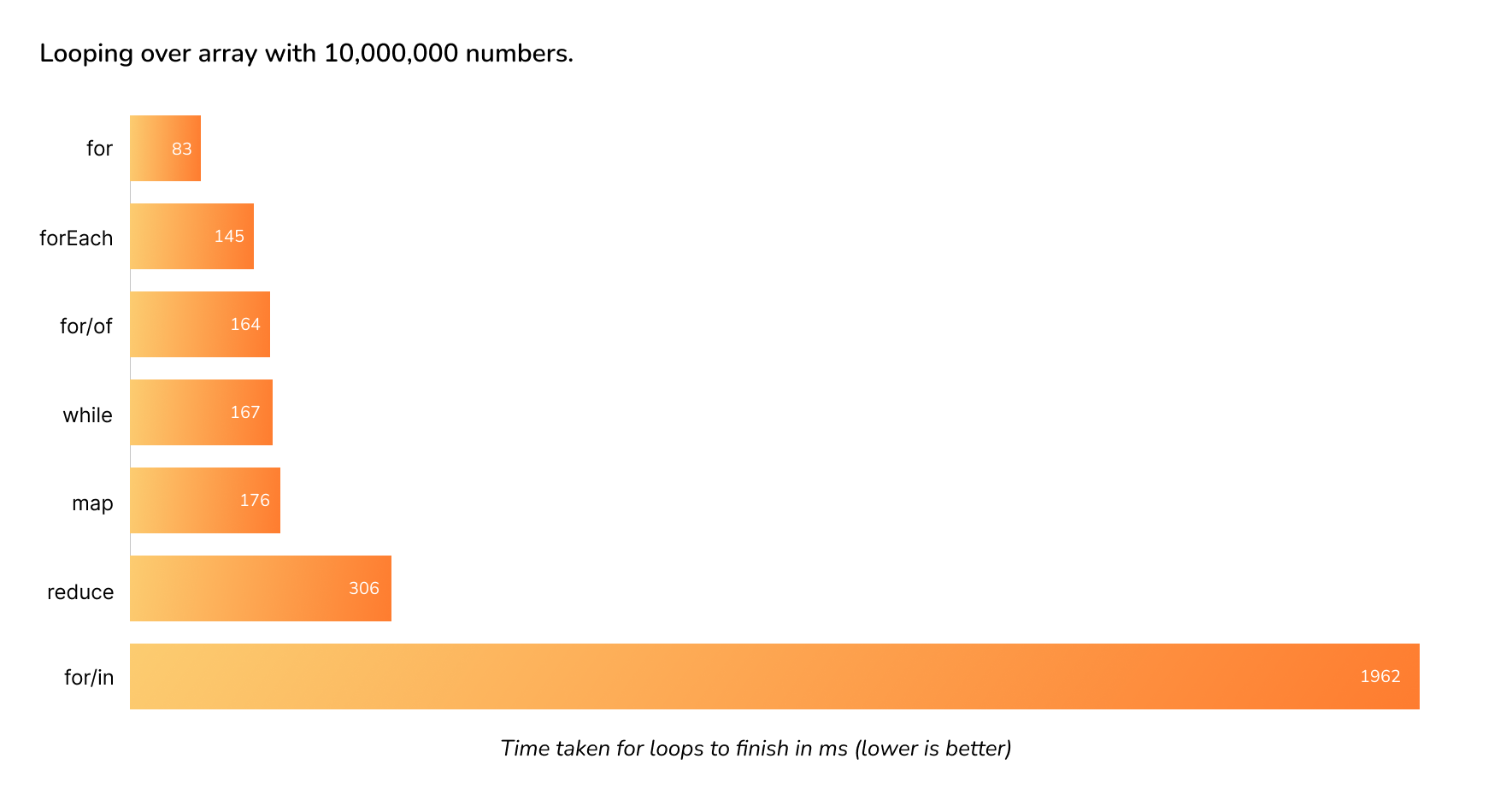
TL;DR
for
loop is fastest
Code
Code is pretty simple and straightforward. I created an array with 10000000
length and filled it in with zeros.
const arr = new Array(10000000).fill(0);
After that I created function for every loop that iterates over items, and pushes current item to array. performance
API is used for
measuring performance, since it is most accurate. Here is an example for for
loop
let resFor = [];
const startFor = performance.now();
for (let i = 0; i < arr?.length; i++) {
resFor.push(i);
}
const endFor = performance.now();
console.log("For loop: ", endFor - startFor);
Hardware and testing
I am using MacBook Pro 13-inch M1 2020 for testing. Every function is running for 5 times, and average value is taken from all those runs.
Notes
As you can see on the graph, for
loop is the fastest, but only on big array of items. I tested same code on 100
, 1000
, and 10000
items, and performance
difference wasn't big at all, which means that on smaller arrays (up to 10000 items) there isn't much reason to optimize loops.
Don't take these tests as only source of truth. Results can and will vary based on you hardware, data that you are looping over and others, but for
loop will be
fastest in most cases.
More in this category
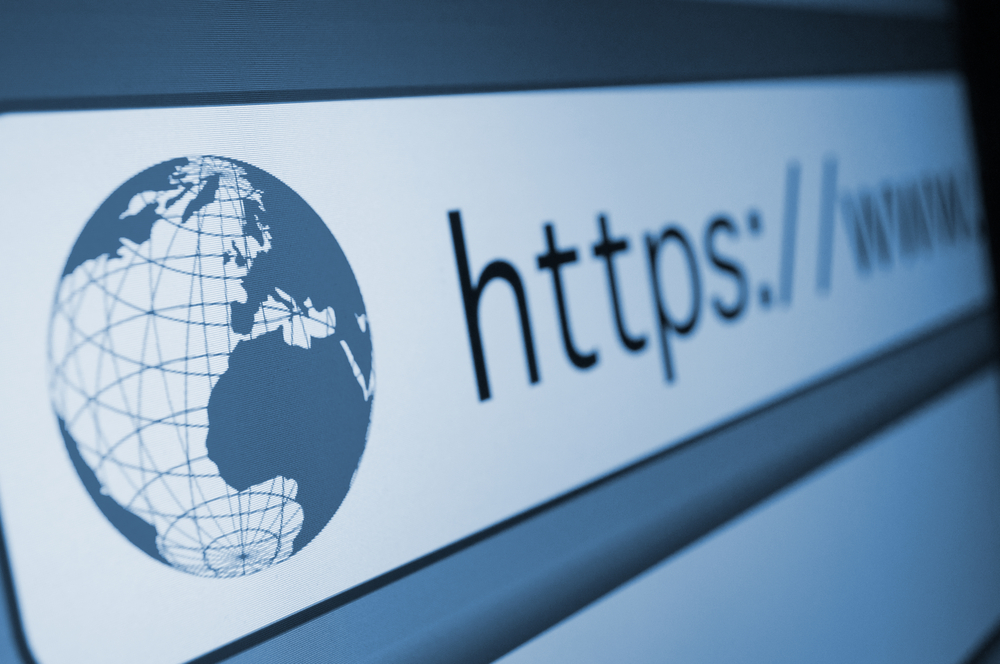
Get any website favicon using free Google API
Google has free API endpoint that allows us to get favicon of any website in different sizes
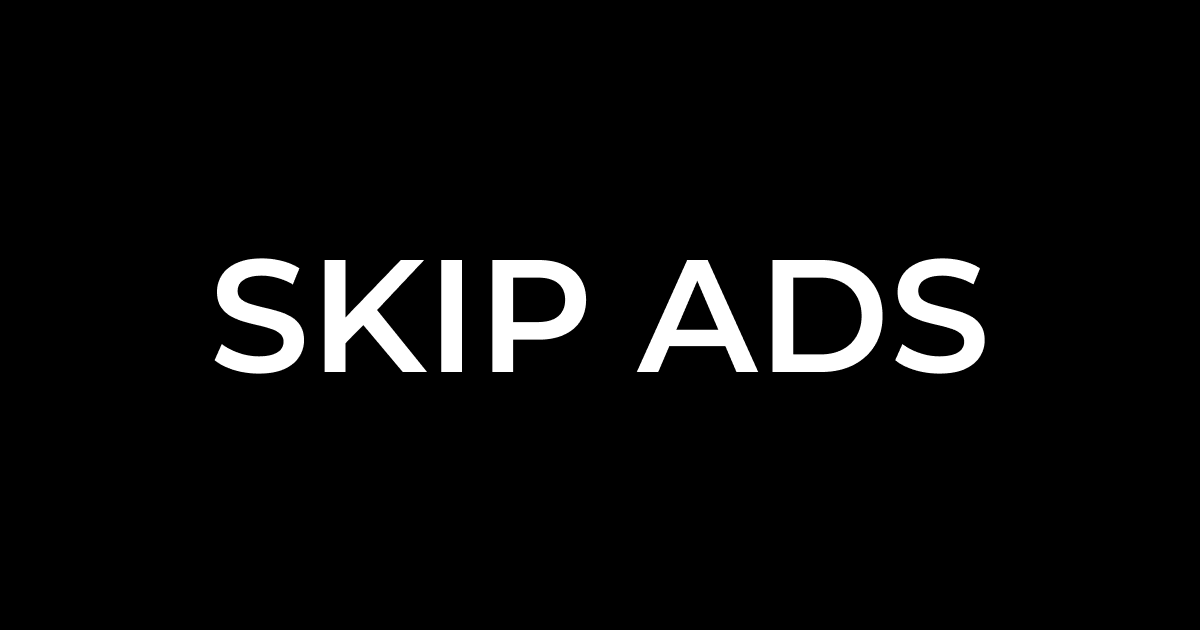
Get rid of YouTube unskippable ADS with simple JavaScript
If you hate waiting 5 seconds to skip YouTube ads, let's fix that with few lines of JavaScript code.
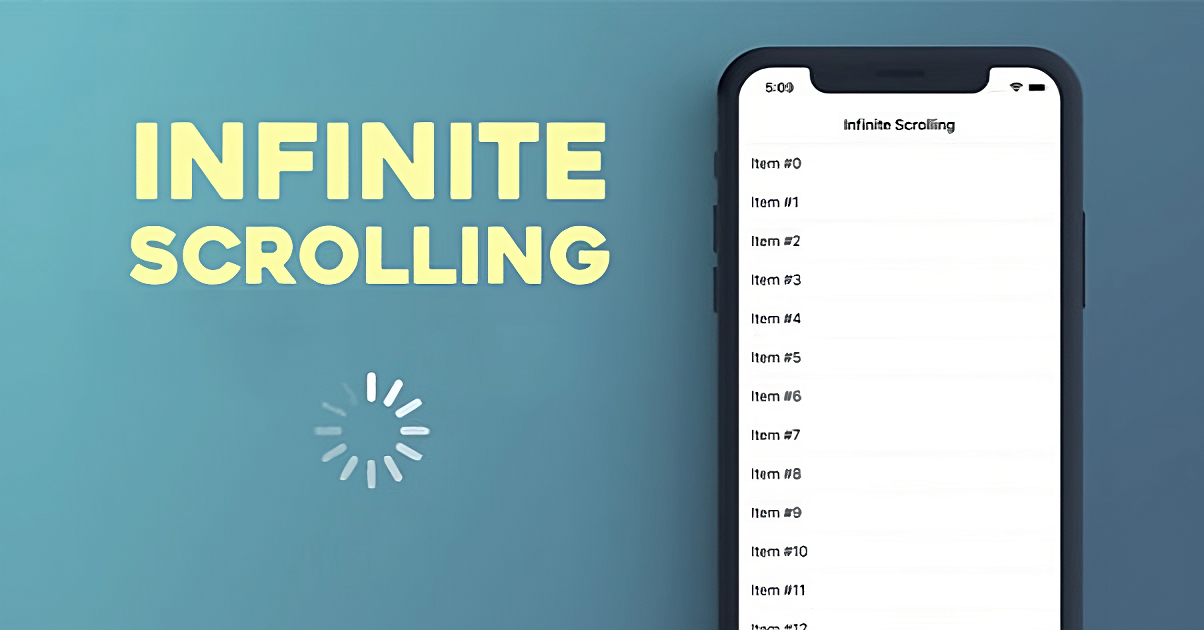
Infinite scroll component in React and NextJS
Infinite scroll is a great way to load huge lists as it provides a great UX for user when compared to traditional pagination
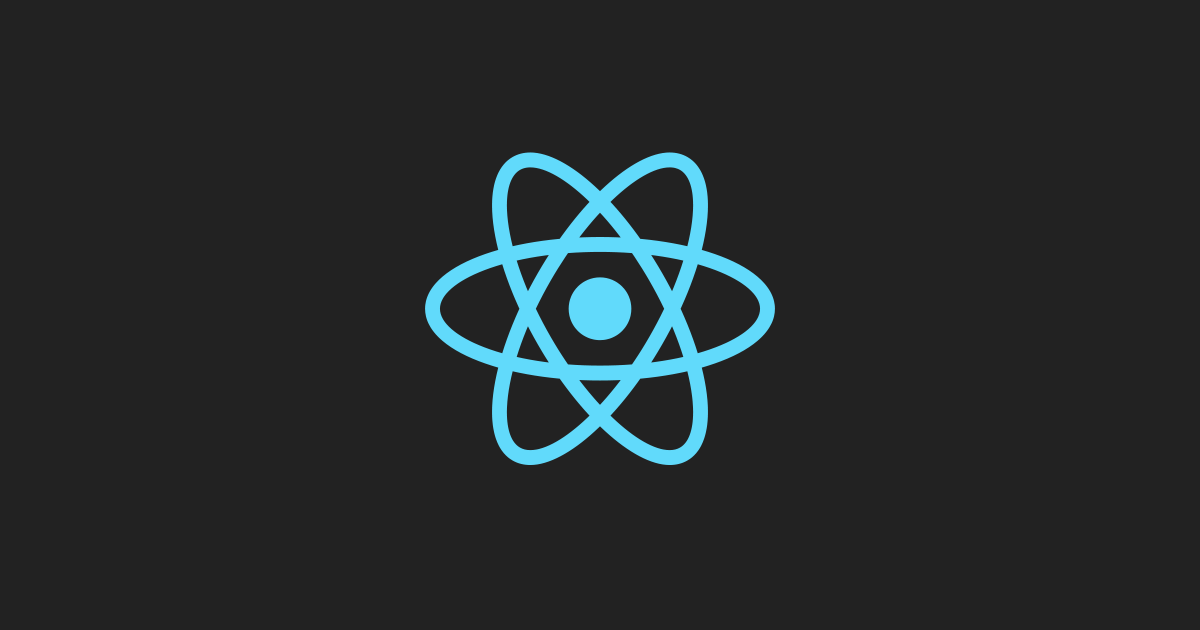
React: Pitch & anti-pitch
Weighing the Advantages and Disadvantages of React for Modern Web Development.