Get rid of YouTube unskippable ADS with simple JavaScript
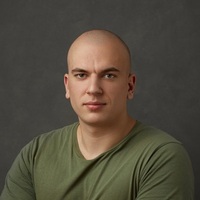
Mileta Dulovic
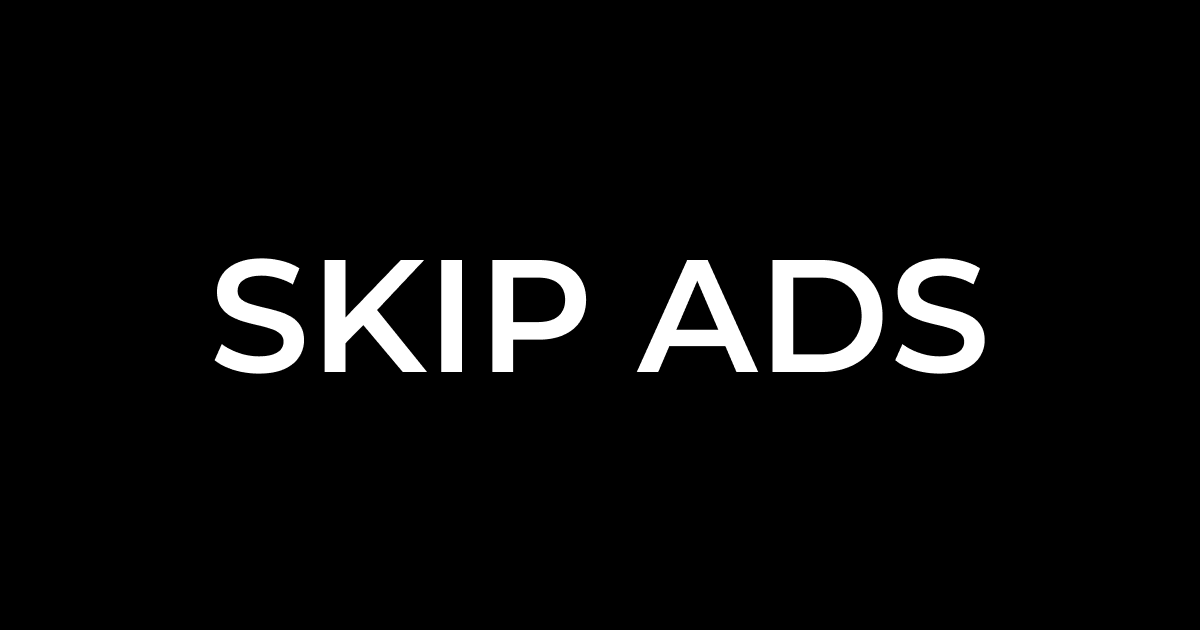
If you want to know how this method works keep reading. If you only came for the code, scroll down to the end of page.
Ads on YouTube
If you are watching YouTube, chances are that you came across ads where you have to wait 5 seconds for the 'Skip Ad' button to be enabled,
end then click it to watch the video. And then same process happens with another video, or in the middle of the video, and it is troublesome.
Ads are just a <video>
tag, and there is a fun little thing about it that we are going to "exploit" today.
HTMLMediaElement
Video
is just another HTMLMediaElement
that we can work with using JavaScript
. If you want to learn more about available methods and properties
click here to read about it on MDN
The HTMLMediaElement interface adds to HTMLElement the properties and methods needed to support basic media-related capabilities that are common to audio and video.
We can manipulate video
element by using available properties, but we are only interested in 2 of them :
HTMLMediaElement.currentTime
HTMLMediaElement.duration
We will set currentTime
of the AD to the duration
of that same AD. This will make video player go to the end of the AD, and 'Skip Ad' button will appear.
Then we will use JavaScript
to automatically click the button and therefore skip the ad.
Code
const stopYoutubeAd = () => {
const ad = document.querySelector('.ad-showing');
if (ad) {
const video = document.querySelector('video');
if (video) {
video.currentTime = video.duration;
setTimeout(() => {
const skipButtons = document.querySelectorAll(".ytp-ad-skip-button");
for (const skipButton of skipButtons) {
skipButton.click();
}
}, 10)
}
}
const overlayAds = document.querySelectorAll(".ytp-ad-overlay-slot");
for (const overlayAd of overlayAds) {
overlayAd.style.visibility = "hidden";
}
}
setInterval(() => {
stopYoutubeAd()
}, 200)
This will make our code run every 200 miliseconds
, so that even if ad is in the middle of the video, it will still work correctly.
If anything is unclear in this code, read the comments I wrote.
Conclusion
You can run this code directly in the console, or you can create a browser extension that works in background, click here to read more about making one. Either way, it is pretty easy and simple to understand, yet really powerful and handy.
More in this category
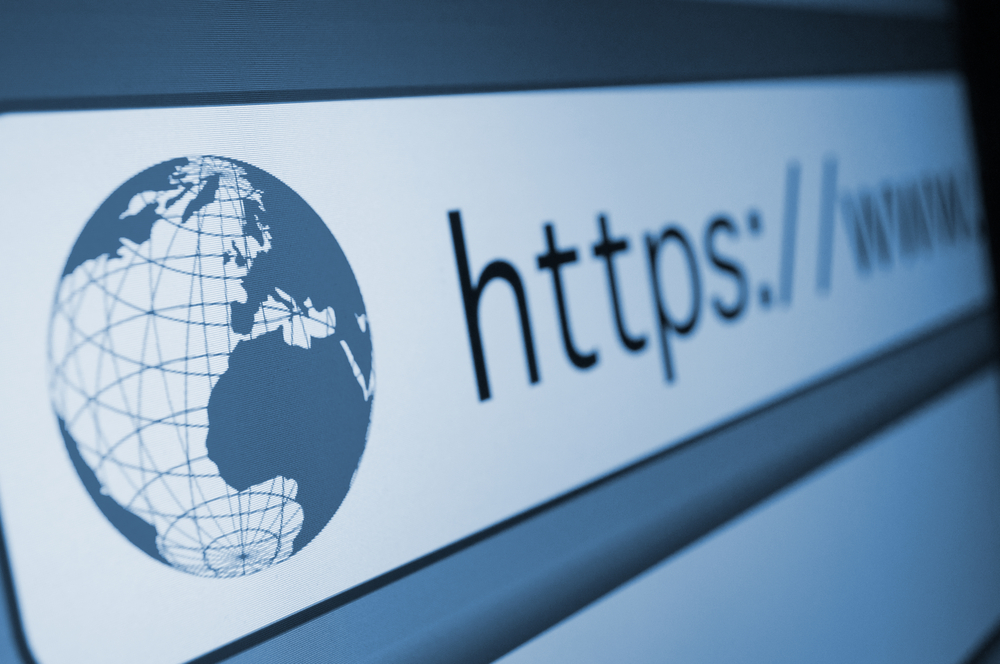
Get any website favicon using free Google API
Google has free API endpoint that allows us to get favicon of any website in different sizes
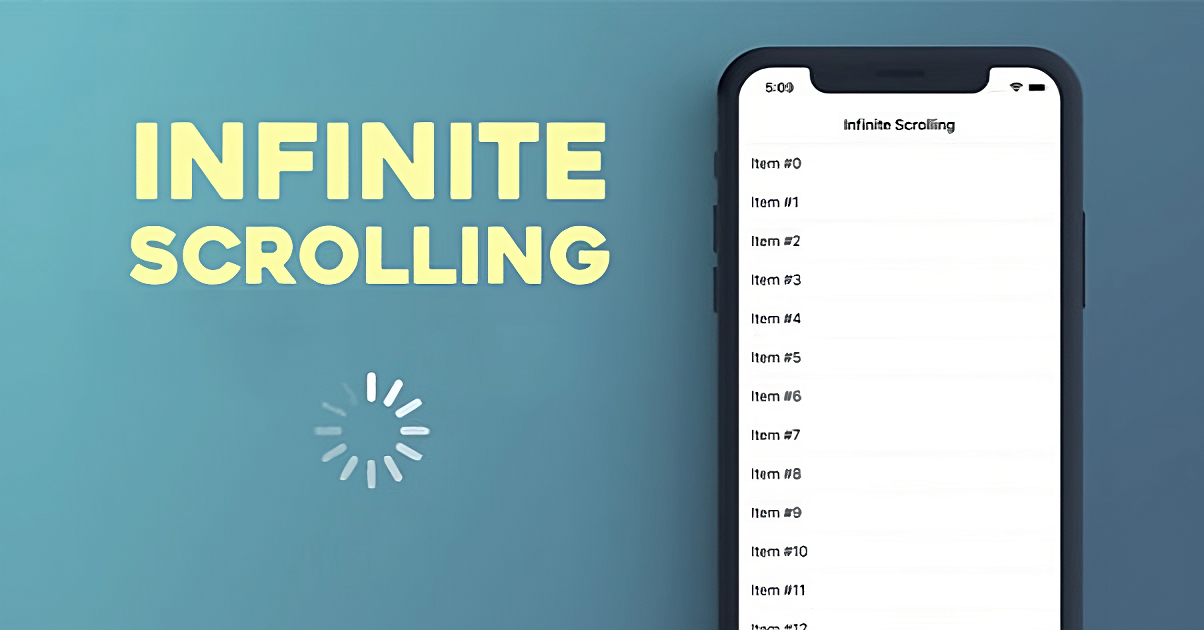
Infinite scroll component in React and NextJS
Infinite scroll is a great way to load huge lists as it provides a great UX for user when compared to traditional pagination
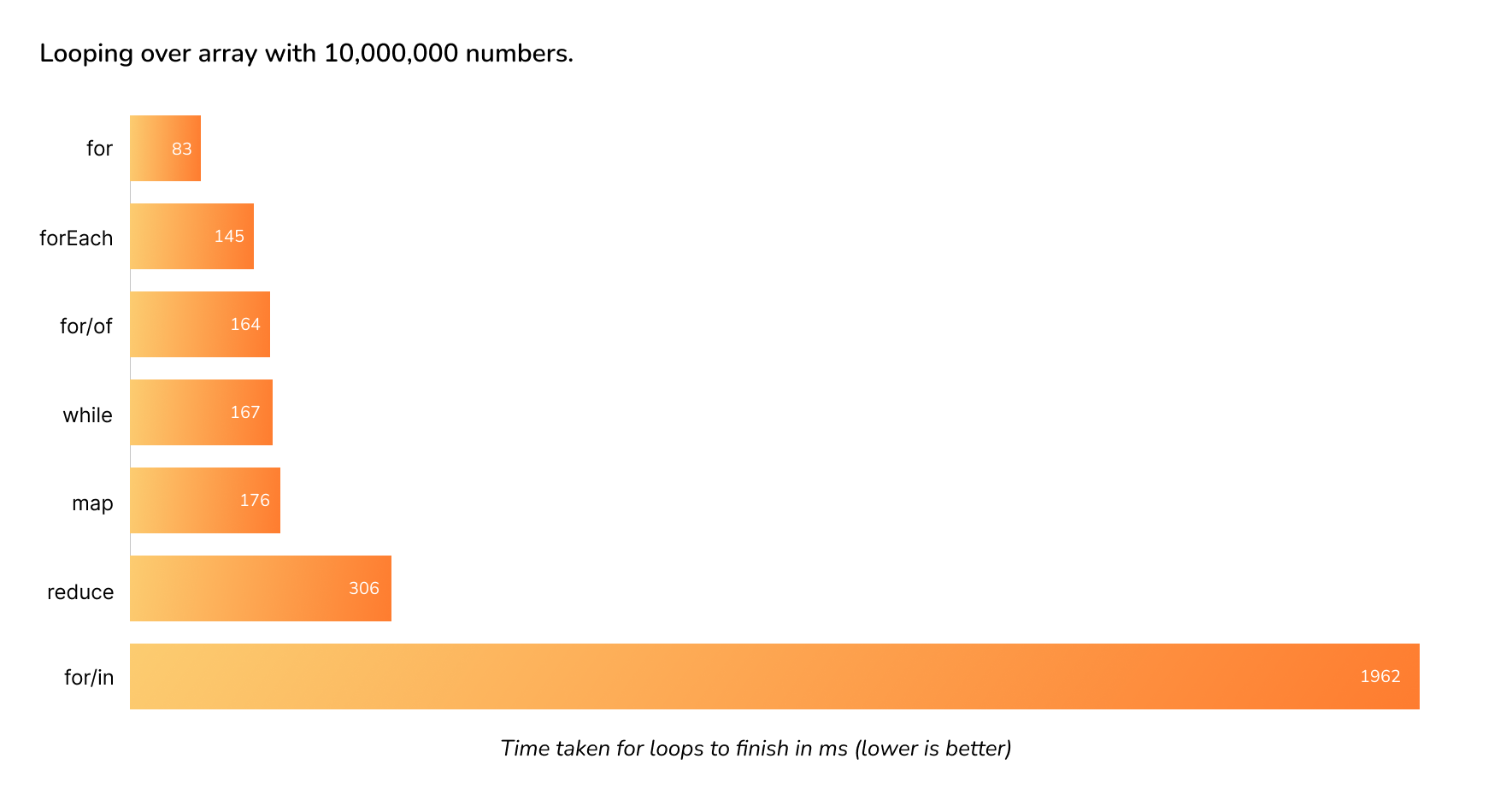
JavaScript Loops performance
Yet another post that iterates over items to measure performance of different loops in JavaScript.
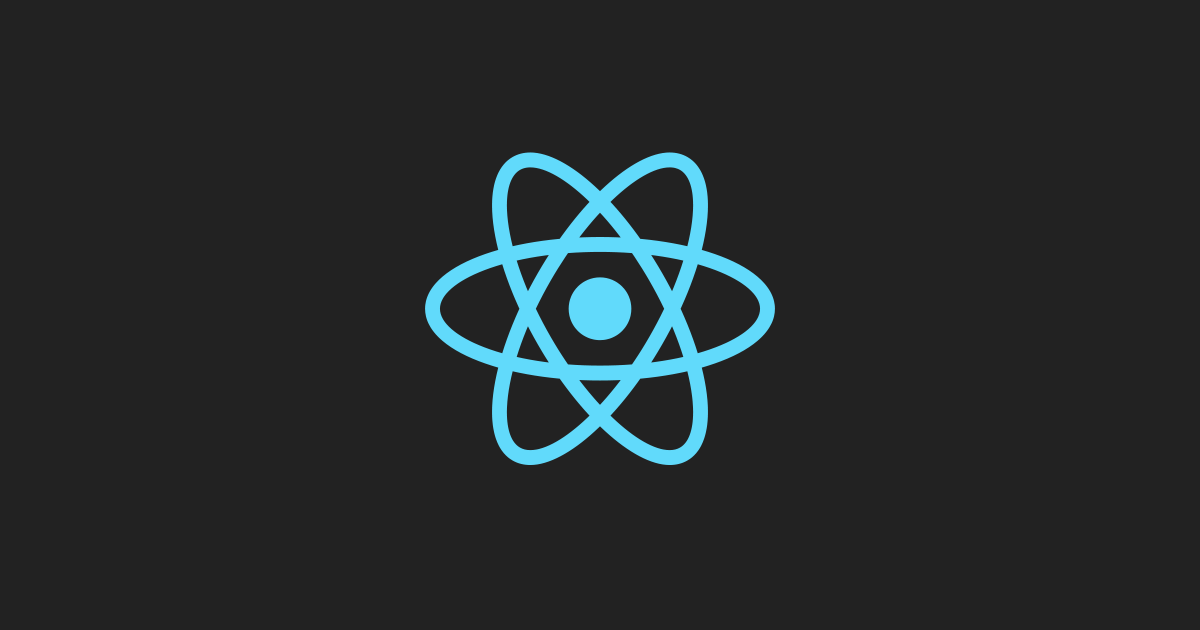
React: Pitch & anti-pitch
Weighing the Advantages and Disadvantages of React for Modern Web Development.